Delay UI Elements in iOS
In my iOS app for the front reception desk I provide a staff sign in/out facility for staff visiting another campus site. The theory is that anyone who works at Dumfries campus visiting Stranraer will sign in via the iPad.
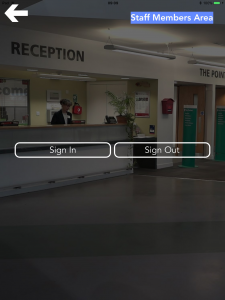
The problem is that there are 200+ records to be loaded from web service on the screen with the sign in / sign out buttons. It is possible if you are very fast to attempt to load one of the other view controllers (sign out list for instance) while the staff CoreData model is being populated (on a new calendar day) or updated. This causes the program to crash as the context of a CoreData object is not allowed to change while it is being mutated.
The solution is to delay the presentation of the buttons until a decent amount of data has been loaded.
As usual I found a great discussion on Stack Overflow about pausing the main thread upon certain conditions.
I have implemented this as follows (NOTE: staffusers is an object – the result of an NSFetchRequest). The sign in and sign out buttons are both hidden by default and the activity spinner is shown by default.
DispatchQueue.main.asyncAfter(deadline: .now() + 1) {
if((staffusers?.count)! < 200) {
sleep(2)
}
else
{
self.btnSignIn.isHidden = false
self.btnSignOut.isHidden = false
self.aiActivity.isHidden = true
}
}
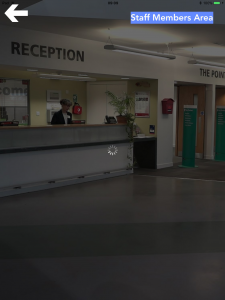
After 1 second, If there are fewer than 200 records in the staffList object the thread will sleep for 2 seconds as a safe enough time span to finish loading but not so long that you would get bored waiting.
As you can see from the code, once the 2 seconds is up the spinner is hidden and the sign in / out buttons are shown.
I can make further safety checks on the number of records present when touching a button but I have not had the need to do this yet.